In this tutorial, we will learn how to create multiple Spring boot microservices and how to use RestTemplate class to make Synchronous communication between multiple microservices.
There are two styles of Microservices Communications:
- Synchronous Communication
- Asynchronous Communication
Synchronous Communication
Asynchronous Communication
What we will Build?
Creating DepartmentService Microservice
1. Create and setup spring boot project (department-service) in IntelliJ IDEA
Let's create a Spring boot project using the spring initializr.
Refer to the below screenshot to enter details while creating the spring boot application using the spring initializr:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>net.javaguides</groupId>
<artifactId>department-service</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>department-service</name>
<description>department-service</description>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
DepartmentService - Configure MySQL Database
spring.datasource.url=jdbc:mysql://localhost:3306/department_db
spring.datasource.username=root
spring.datasource.password=Mysql@123
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQLDialect
spring.jpa.hibernate.ddl-auto=update
DepartmentService - Create Department JPA Entity
package net.javaguides.departmentservice.entity;
import javax.persistence.*;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
@Entity
@Table(name = "departments")
@NoArgsConstructor
@AllArgsConstructor
@Setter
@Getter
public class Department {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String departmentName;
private String departmentAddress;
private String departmentCode;
}
DepartmentService - Create Spring Data JPA Repository
package net.javaguides.departmentservice.repository;
import net.javaguides.departmentservice.entity.Department;
import org.springframework.data.jpa.repository.JpaRepository;
public interface DepartmentRepository extends JpaRepository<Department, Long> {
}
DepartmentService - Create Service Layer
DepartmentService Interface
package net.javaguides.departmentservice.service;
import net.javaguides.departmentservice.entity.Department;
public interface DepartmentService {
Department saveDepartment(Department department);
Department getDepartmentById(Long departmentId);
}
DepartmentServiceImpl class
package net.javaguides.departmentservice.service.impl;
import lombok.AllArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import net.javaguides.departmentservice.entity.Department;
import net.javaguides.departmentservice.repository.DepartmentRepository;
import net.javaguides.departmentservice.service.DepartmentService;
import org.springframework.stereotype.Service;
@Service
@AllArgsConstructor
@Slf4j
public class DepartmentServiceImpl implements DepartmentService {
private DepartmentRepository departmentRepository;
@Override
public Department saveDepartment(Department department) {
return departmentRepository.save(department);
}
@Override
public Department getDepartmentById(Long departmentId) {
return departmentRepository.findById(departmentId).get();
}
}
DepartmentService - Create Controller Layer: DepartmentController
package net.javaguides.departmentservice.controller;
import lombok.AllArgsConstructor;
import net.javaguides.departmentservice.entity.Department;
import net.javaguides.departmentservice.service.DepartmentService;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("api/departments")
@AllArgsConstructor
public class DepartmentController {
private DepartmentService departmentService;
@PostMapping
public ResponseEntity<Department> saveDepartment(@RequestBody Department department){
Department savedDepartment = departmentService.saveDepartment(department);
return new ResponseEntity<>(savedDepartment, HttpStatus.CREATED);
}
@GetMapping("{id}")
public ResponseEntity<Department> getDepartmentById(@PathVariable("id") Long departmentId){
Department department = departmentService.getDepartmentById(departmentId);
return ResponseEntity.ok(department);
}
}
DepartmentService - Start Spring Boot Application
$ mvn spring-boot:run
DepartmentService - Test REST APIs using Postman Client
Save Department REST API:
Get Single Department REST API:
2. Creating UserService Microservice
1. Create and setup spring boot project (user-service) in IntelliJ IDEA
Let's create a Spring boot project using the spring initializr.
Refer to the below screenshot to enter details while creating the spring boot application using the spring initializr:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>net.javaguides</groupId>
<artifactId>user-service</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>user-service</name>
<description>user-service</description>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
UserService - Configure MySQL Database
spring.datasource.url=jdbc:mysql://localhost:3306/employee_db
spring.datasource.username=root
spring.datasource.password=Mysql@123
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQLDialect
spring.jpa.hibernate.ddl-auto=update
UserService - Change the Server Port
server.port = 8081
UserService - Create User JPA Entity
package net.javaguides.userservice.entity;
import javax.persistence.*;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
@Entity
@Table(name = "users")
@Setter
@Getter
@NoArgsConstructor
@AllArgsConstructor
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String firstName;
private String lastName;
@Column(nullable = false, unique = true)
private String email;
private String departmentId;
}
UserService - Create Spring Data JPA Repository
package net.javaguides.userservice.repository;
import net.javaguides.userservice.entity.User;
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
}
UserService - Create DTO Classes
DepartmentDto
package net.javaguides.userservice.dto;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
@Setter
@Getter
@AllArgsConstructor
@NoArgsConstructor
public class DepartmentDto {
private Long id;
private String departmentName;
private String departmentAddress;
private String departmentCode;
}
UserDto
package net.javaguides.userservice.dto;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
@Setter
@Getter
@NoArgsConstructor
@AllArgsConstructor
public class UserDto {
private Long id;
private String firstName;
private String lastName;
private String email;
}
ResponseDto
package net.javaguides.userservice.dto;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
@Setter
@Getter
@NoArgsConstructor
@AllArgsConstructor
public class ResponseDto {
private DepartmentDto department;
private UserDto user;
}
UserService - Configure RestTemplate as Spring Bean
package net.javaguides.userservice;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.web.client.RestTemplate;
@SpringBootApplication
public class UserServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserServiceApplication.class, args);
}
@Bean
public RestTemplate restTemplate(){
return new RestTemplate();
}
}
UserService - Create Service Layer
UserService Interface
package net.javaguides.userservice.service;
import net.javaguides.userservice.dto.ResponseDto;
import net.javaguides.userservice.entity.User;
public interface UserService {
User saveUser(User user);
ResponseDto getUser(Long userId);
}
UserServiceImpl class
package net.javaguides.userservice.service.impl;
import lombok.AllArgsConstructor;
import net.javaguides.userservice.dto.DepartmentDto;
import net.javaguides.userservice.dto.ResponseDto;
import net.javaguides.userservice.dto.UserDto;
import net.javaguides.userservice.entity.User;
import net.javaguides.userservice.repository.UserRepository;
import net.javaguides.userservice.service.UserService;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
@Service
@AllArgsConstructor
public class UserServiceImpl implements UserService {
private UserRepository userRepository;
private RestTemplate restTemplate;
@Override
public User saveUser(User user) {
return userRepository.save(user);
}
@Override
public ResponseDto getUser(Long userId) {
ResponseDto responseDto = new ResponseDto();
User user = userRepository.findById(userId).get();
UserDto userDto = mapToUser(user);
ResponseEntity<DepartmentDto> responseEntity = restTemplate
.getForEntity("http://localhost:8080/api/departments/" + user.getDepartmentId(),
DepartmentDto.class);
DepartmentDto departmentDto = responseEntity.getBody();
System.out.println(responseEntity.getStatusCode());
responseDto.setUser(userDto);
responseDto.setDepartment(departmentDto);
return responseDto;
}
private UserDto mapToUser(User user){
UserDto userDto = new UserDto();
userDto.setId(user.getId());
userDto.setFirstName(user.getFirstName());
userDto.setLastName(user.getLastName());
userDto.setEmail(user.getEmail());
return userDto;
}
}
ResponseEntity<DepartmentDto> responseEntity = restTemplate
.getForEntity("http://localhost:8080/api/departments/" + user.getDepartmentId(),
DepartmentDto.class);
UserService - Create Controller Layer: UserController
package net.javaguides.userservice.controller;
import lombok.AllArgsConstructor;
import net.javaguides.userservice.dto.ResponseDto;
import net.javaguides.userservice.entity.User;
import net.javaguides.userservice.service.UserService;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("api/users")
@AllArgsConstructor
public class UserController {
private UserService userService;
@PostMapping
public ResponseEntity<User> saveUser(@RequestBody User user){
User savedUser = userService.saveUser(user);
return new ResponseEntity<>(savedUser, HttpStatus.CREATED);
}
@GetMapping("{id}")
public ResponseEntity<ResponseDto> getUser(@PathVariable("id") Long userId){
ResponseDto responseDto = userService.getUser(userId);
return ResponseEntity.ok(responseDto);
}
}
UserService - Start Spring Boot Application
$ mvn spring-boot:run
UserService - Test REST APIs using Postman Client
Save User REST API:
Get User REST API: 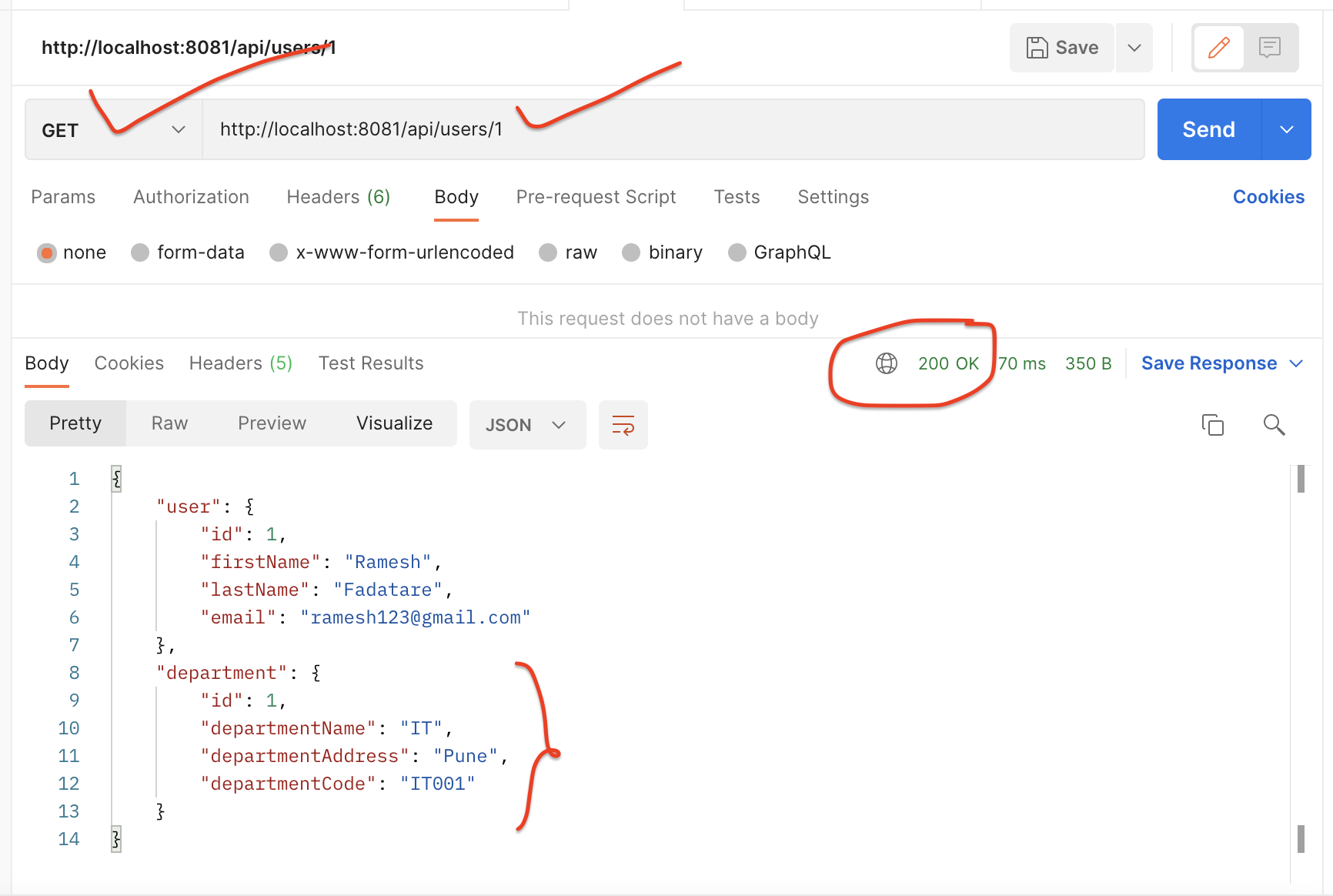
Conclusion
Related Tutorials
✅ Spring Boot Microservices Communication Example using RestTemplate
✅ Spring Boot Microservices Communication Example using WebClient
✅ Spring Boot Microservices Communication Example using Spring Cloud Open Feign
✅ Spring Boot Microservices - Spring Cloud Config Server
✅ Spring Boot Microservices - Spring Cloud Netflix Eureka-based Service Registry
Comments
Post a Comment
Leave Comment