In this tutorial, we will discuss how to integrate the Oracle database with the Spring boot application. We will develop CRUD Rest web services using Spring boot with Oracle database and we will test it using Postman Client.
Let's develop a complete CRUD RESTFul APIs for a simple Employee Management System using Spring Boot, JPA/Hibernate, and Oracle database.
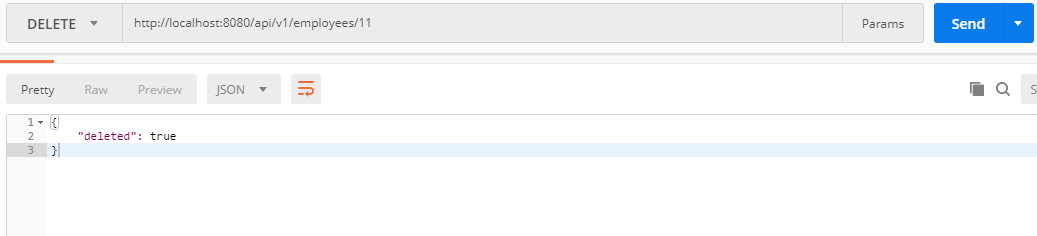
Spring Boot CRUD Restful API with Oracle database
Quick Steps to Configure Spring Boot to use Oracle database
It's very easy to configure Spring Boot to use the Oracle database. We are using Hibernate so which will support out of the box to work with different database vendors without changing underlying code.
Follow below two steps to configure Oracle in the Spring boot application:
Step 1: Provide Oracle dependency in your pom.xml file:
<!-- https://mvnrepository.com/artifact/com.oracle.jdbc/ojdbc8 --> <dependency> <groupId>com.oracle.jdbc</groupId> <artifactId>ojdbc8</artifactId> <version>12.2.0.1</version> </dependency>
Step 2: Let’s configure Spring Boot to use Oracle as our data source. We are simply adding Oracle database URL, username, and password in the src/main/resources/application.properties file -
# create and drop tables spring.jpa.hibernate.ddl-auto=create-drop # Oracle settings spring.datasource.url=jdbc:oracle:thin:@localhost:1521:xe spring.datasource.username=system spring.datasource.password=password spring.datasource.driver-class-name=oracle.jdbc.driver.OracleDriver
That's all. Now you are good to go.
Let's develop a complete CRUD RESTFul APIs for a simple Employee Management System using Spring Boot, JPA/Hibernate, and Oracle database.
Tools and technologies used:
- Spring Boot - 2.0.4.RELEASE
- JDK - 1.8 or later
- Spring Framework - 5.0.8 RELEASE
- Hibernate - 5.2.17.Final
- Spring Data JPA
- Oracle database 11g express
- Oracle JDBC driver ojdbc8.jar
- Maven - 3.2+
- IDE - Eclipse or Spring Tool Suite (STS)
What we'll build
We will build CRUD RESTFul APIs for a Simple Employee Management System using Spring Boot and the Oracle database. Following are five REST APIs (Controller handler methods) created for Employee resource.
1. Creating and Importing a Project
There are many ways to create a Spring Boot application. You can refer below articles to create a Spring Boot application.
>> Create Spring Boot Project With Spring Initializer
>> Create Spring Boot Project in Spring Tool Suite [STS]
>> Create Spring Boot Project in Spring Tool Suite [STS]
Refer project structure or packaging structure in the next step.
2. Packaging Structure
Following is the packing structure of our Employee Management System -
3. The pom.xml File
You will need to add the Oracle Driver to your Maven dependencies:
<!-- https://mvnrepository.com/artifact/com.oracle.jdbc/ojdbc8 -->
<dependency>
<groupId>com.oracle.jdbc</groupId>
<artifactId>ojdbc8</artifactId>
<version>12.2.0.1</version>
</dependency>
Here is the complete maven pom.xml file:
<?xml version="1.0" encoding="UTF-8"?>
<project
xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>net.guides.springboot2</groupId>
<artifactId>springboot2-jpa-crud-example</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>springboot2-jpa-crud-example</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
<relativePath />
<!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/com.oracle.jdbc/ojdbc8 -->
<dependency>
<groupId>com.oracle.jdbc</groupId>
<artifactId>ojdbc8</artifactId>
<version>12.2.0.1</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
4. Oracle Database Configuration
Let's configure the Oracle data source and hibernate properties:
# create and drop tables
spring.jpa.hibernate.ddl-auto=create-drop
# Oracle settings
spring.datasource.url=jdbc:oracle:thin:@localhost:1521:xe
spring.datasource.username=system
spring.datasource.password=password
spring.datasource.driver-class-name=oracle.jdbc.driver.OracleDriver
5. Create JPA Entity - Employee.java
package net.guides.springboot2.springboot2jpacrudexample.model; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name = "employees") public class Employee { private long id; private String firstName; private String lastName; private String emailId; public Employee() { } public Employee(String firstName, String lastName, String emailId) { this.firstName = firstName; this.lastName = lastName; this.emailId = emailId; } @Id @GeneratedValue(strategy = GenerationType.AUTO) public long getId() { return id; } public void setId(long id) { this.id = id; } @Column(name = "first_name", nullable = false) public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } @Column(name = "last_name", nullable = false) public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } @Column(name = "email_address", nullable = false) public String getEmailId() { return emailId; } public void setEmailId(String emailId) { this.emailId = emailId; } }
6. Create a Spring Data Repository - EmployeeRepository.java
package net.guides.springboot2.springboot2jpacrudexample.repository; import org.springframework.data.jpa.repository.JpaRepository; import org.springframework.stereotype.Repository; import net.guides.springboot2.springboot2jpacrudexample.model.Employee; @Repository public interface EmployeeRepository extends JpaRepository<Employee, Long>{ }
7. Create Spring Rest Controller - EmployeeController.java
package net.guides.springboot2.springboot2jpacrudexample.controller; import java.util.HashMap; import java.util.List; import java.util.Map; import javax.validation.Valid; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.ResponseEntity; import org.springframework.web.bind.annotation.CrossOrigin; import org.springframework.web.bind.annotation.DeleteMapping; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.PutMapping; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import net.guides.springboot2.springboot2jpacrudexample.exception.ResourceNotFoundException; import net.guides.springboot2.springboot2jpacrudexample.model.Employee; import net.guides.springboot2.springboot2jpacrudexample.repository.EmployeeRepository; @RestController @RequestMapping("/api/v1") public class EmployeeController { @Autowired private EmployeeRepository employeeRepository; @GetMapping("/employees") public List < Employee > getAllEmployees() { return employeeRepository.findAll(); } @GetMapping("/employees/{id}") public ResponseEntity < Employee > getEmployeeById(@PathVariable(value = "id") Long employeeId) throws ResourceNotFoundException { Employee employee = employeeRepository.findById(employeeId) .orElseThrow(() -> new ResourceNotFoundException("Employee not found for this id :: " + employeeId)); return ResponseEntity.ok().body(employee); } @PostMapping("/employees") public Employee createEmployee(@Valid @RequestBody Employee employee) { return employeeRepository.save(employee); } @PutMapping("/employees/{id}") public ResponseEntity < Employee > updateEmployee(@PathVariable(value = "id") Long employeeId, @Valid @RequestBody Employee employeeDetails) throws ResourceNotFoundException { Employee employee = employeeRepository.findById(employeeId) .orElseThrow(() -> new ResourceNotFoundException("Employee not found for this id :: " + employeeId)); employee.setEmailId(employeeDetails.getEmailId()); employee.setLastName(employeeDetails.getLastName()); employee.setFirstName(employeeDetails.getFirstName()); final Employee updatedEmployee = employeeRepository.save(employee); return ResponseEntity.ok(updatedEmployee); } @DeleteMapping("/employees/{id}") public Map < String, Boolean > deleteEmployee(@PathVariable(value = "id") Long employeeId) throws ResourceNotFoundException { Employee employee = employeeRepository.findById(employeeId) .orElseThrow(() -> new ResourceNotFoundException("Employee not found for this id :: " + employeeId)); employeeRepository.delete(employee); Map < String, Boolean > response = new HashMap < > (); response.put("deleted", Boolean.TRUE); return response; } }
8. Exception(Error) Handling for RESTful Services
Spring Boot provides a good default implementation for exception handling for RESTful Services. Let’s quickly look at the default Exception Handling features provided by Spring Boot.
Resource Not Present
Here's what happens when you fire a request to not resource found: http://localhost:8080/some-dummy-url
{
"timestamp": 1512713804164,
"status": 404,
"error": "Not Found",
"message": "No message available",
"path": "/some-dummy-url"
}
That's a cool error response. It contains all the details that are typically needed.
What happens when we throw an Exception?
Let’s see what Spring Boot does when an exception is thrown from a Resource. we can specify the Response Status for a specific exception along with the definition of the Exception of the @ResponseStatus annotation.
Let's create a ResourceNotFoundException.java class.
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ResponseStatus;
@ResponseStatus(value = HttpStatus.NOT_FOUND)
public class ResourceNotFoundException extends Exception{
private static final long serialVersionUID = 1L;
public ResourceNotFoundException(String message){
super(message);
}
}
Customizing Error Response Structure
The default error response provided by Spring Boot contains all the details that are typically needed.
However, you might want to create a framework independent response structure for your organization. In that case, you can define a specific error response structure.
Let’s define a simple error response bean.
import java.util.Date;
public class ErrorDetails {
private Date timestamp;
private String message;
private String details;
public ErrorDetails(Date timestamp, String message, String details) {
super();
this.timestamp = timestamp;
this.message = message;
this.details = details;
}
public Date getTimestamp() {
return timestamp;
}
public String getMessage() {
return message;
}
public String getDetails() {
return details;
}
}
To use ErrorDetails to return the error response, let’s create a GlobalExceptionHandler class annotated with @ControllerAdvice annotation. This class handles exception-specific and global exceptions in a single place.
import java.util.Date;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.context.request.WebRequest;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<?> resourceNotFoundException(ResourceNotFoundException ex, WebRequest request) {
ErrorDetails errorDetails = new ErrorDetails(new Date(), ex.getMessage(), request.getDescription(false));
return new ResponseEntity<>(errorDetails, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<?> globleExcpetionHandler(Exception ex, WebRequest request) {
ErrorDetails errorDetails = new ErrorDetails(new Date(), ex.getMessage(), request.getDescription(false));
return new ResponseEntity<>(errorDetails, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
9. Running Application
This spring boot application has an entry point Java class called Application.java with the public static void main(String[] args) method, which you can run to start the application.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
@SpringBootApplication is a convenience annotation that adds all of the following:
- @Configuration tags the class as a source of bean definitions for the application context.
- @EnableAutoConfiguration tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings.
- Normally you would add @EnableWebMvc for a Spring MVC app, but Spring Boot adds it automatically when it sees spring-webmvc on the classpath. This flags the application as a web application and activates key behaviors such as setting up a DispatcherServlet.
- @ComponentScan tells Spring to look for other components, configurations, and services in the hello package, allowing it to find the controllers.
The main() method uses Spring Boot’s SpringApplication.run() method to launch an application.
There is a separate beautiful article for integration testing for REST APIs on:
>> Spring Boot 2 REST APIs Integration Testing
10. Integration Testing for REST APIs
There is a separate beautiful article for integration testing for REST APIs on:
>> Spring Boot 2 REST APIs Integration Testing
11. Testing REST APIs via Postman Client
1. Create Employee REST API
HTTP Method: POST
Request URL: http://localhost:8080/api/v1/employees
Note that request and response JSON in the above diagram, the response contains database auto-generated id.
2. Get Employee by ID REST API
HTTP Method: GET
Request URL: http://localhost:8080/api/v1/employees/11
5. Delete Employee REST API
HTTP Method: DELETE
Request URL: http://localhost:8080/api/v1/employees/11
once i kill the local server, the table doesnt exist only in the db. How is that possible?
ReplyDeletei can build successfully but when i want to run it, its throwing classnotfoundexception io.r2dbc.spi.connectionfactory
ReplyDeletewhere i'm wrong?
Thank you, Great tutorial all facts are correct......
ReplyDelete