In this article, we will show how to use c3p0 connection pooling in Hibernate applications.
This tutorial is upgraded to use Hibernate 6+ and Java 17.
C3p0 is an open-source JDBC connection pooling library, with support for caching and reuse of PreparedStatements.
Hibernate provides support for Java applications to use c3p0 for connection pooling with additional configuration settings.
In this article, I will show you how to configure the c3p0 library with Hibernate ORM framework.
Hibernate c3p0 Connection Pool Complete Step-By-Step Example
Let's start developing step by step Hibernate application using Maven as a project management and build tool.
Technologies and tools used
- Hibernate 6.1.7.Final
- IDE - Eclipse
- Maven 3.5.3
- JavaSE 17
- MySQL - 8.0.32
- c3p0 - 0.9.5.2
Development Steps
- Create a Simple Maven Project
- Project Directory Structure
- Add jar Dependencies to pom.xml
- Creating the JPA Entity Class(Persistent class)
- Hibernate + C3p0 configuration
- Create StudentDao Class
- Create the Main class and Run an Application
1. Create a Simple Maven Project
Use the How to Create a Simple Maven Project in Eclipse article to create a simple Maven project in Eclipse IDE.
2. Project Directory Structure
The project directory structure for your reference -
3. Add jar Dependencies to pom.xml
<project
xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>net.javaguides.hibernate</groupId>
<artifactId>hibernate-tutorial</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<artifactId>hibernate-c3p0-connection-pooling-example</artifactId>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<!-- https://mvnrepository.com/artifact/mysql/mysql-connector-java -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.32</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.hibernate/hibernate-core -->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.1.7.Final</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-c3p0</artifactId>
<version>6.1.7.Final</version>
</dependency>
<!-- c3p0 -->
<dependency>
<groupId>com.mchange</groupId>
<artifactId>c3p0</artifactId>
<version>0.9.5.2</version>
</dependency>
</dependencies>
<build>
<sourceDirectory>src/main/java</sourceDirectory>
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.5.1</version>
<configuration>
<source>17</source>
<target>17</target>
</configuration>
</plugin>
</plugins>
</build>
</project>
To integrate c3p0 connection pooling, we need to add below jar dependencies:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-c3p0</artifactId>
<version>6.1.7.Final</version>
</dependency>
<!-- c3p0 -->
<dependency>
<groupId>com.mchange</groupId>
<artifactId>c3p0</artifactId>
<version>0.9.5.2</version>
</dependency>
4. Creating the JPA Entity Class(Persistent class)
Let's create a Student persistent class that is mapped to a database table.
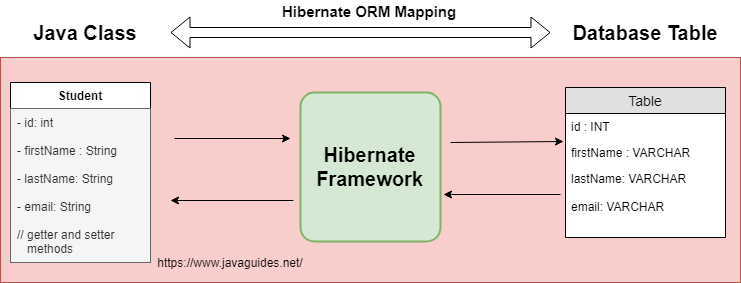
A simple Persistent class should follow some rules:
- A no-arg constructor
- Provide an identifier property
- Declare getter and setter methods:
- Prefer non-final class
Let's create a Student entity class under net.javaguides.hibernate.entity package as follows.
package net.javaguides.hibernate.entity;
import jakarta.persistence.*;
@Entity
@Table(name = "student")
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "id")
private int id;
@Column(name = "first_name")
private String firstName;
@Column(name = "last_name")
private String lastName;
@Column(name = "email")
private String email;
public Student() {
}
public Student(String firstName, String lastName, String email) {
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public String toString() {
return "Student [id=" + id + ", firstName=" + firstName + ", lastName=" + lastName + ", email=" + email + "]";
}
}
5. Hibernate + C3p0 configuration
To enable c3p0 connection pooling, add the following c3p0 configuration settings in HibernateUtil class.
// c3p0 configuration
settings.put(Environment.C3P0_MIN_SIZE, 5); //Minimum size of pool
settings.put(Environment.C3P0_MAX_SIZE, 20); //Maximum size of pool
settings.put(Environment.C3P0_ACQUIRE_INCREMENT, 1); //Number of connections acquired at a time when pool is exhausted
settings.put(Environment.C3P0_TIMEOUT, 1800); //Connection idle time
settings.put(Environment.C3P0_MAX_STATEMENTS, 150); //PreparedStatement cache size
settings.put(Environment.C3P0_CONFIG_PREFIX + ".initialPoolSize", 5);
Here is the complete HibernateUtil class to bootstrap hibernate application.
package net.javaguides.hibernate.util;
import java.util.Properties;
import org.hibernate.SessionFactory;
import org.hibernate.boot.registry.StandardServiceRegistryBuilder;
import org.hibernate.cfg.Configuration;
import org.hibernate.cfg.Environment;
import org.hibernate.service.ServiceRegistry;
import net.javaguides.hibernate.entity.Student;
public class HibernateUtil {
private static SessionFactory sessionFactory;
public static SessionFactory getSessionFactory() {
if (sessionFactory == null) {
try {
Configuration configuration = new Configuration();
// Hibernate settings equivalent to hibernate.cfg.xml's properties
Properties settings = new Properties();
settings.put(Environment.DRIVER, "com.mysql.cj.jdbc.Driver");
settings.put(Environment.URL, "jdbc:mysql://localhost:3306/hibernate_db?useSSL=false");
settings.put(Environment.USER, "root");
settings.put(Environment.PASS, "root");
settings.put(Environment.SHOW_SQL, "true");
settings.put(Environment.CURRENT_SESSION_CONTEXT_CLASS, "thread");
settings.put(Environment.HBM2DDL_AUTO, "create-drop");
// c3p0 configuration
settings.put(Environment.C3P0_MIN_SIZE, 5); //Minimum size of pool
settings.put(Environment.C3P0_MAX_SIZE, 20); //Maximum size of pool
settings.put(Environment.C3P0_ACQUIRE_INCREMENT, 1); //Number of connections acquired at a time when pool is exhausted
settings.put(Environment.C3P0_TIMEOUT, 1800); //Connection idle time
settings.put(Environment.C3P0_MAX_STATEMENTS, 150); //PreparedStatement cache size
settings.put(Environment.C3P0_CONFIG_PREFIX + ".initialPoolSize", 5);
configuration.setProperties(settings);
configuration.addAnnotatedClass(Student.class);
ServiceRegistry serviceRegistry = new StandardServiceRegistryBuilder()
.applySettings(configuration.getProperties()).build();
System.out.println("Hibernate Java Config serviceRegistry created");
sessionFactory = configuration.buildSessionFactory(serviceRegistry);
return sessionFactory;
} catch (Exception e) {
e.printStackTrace();
}
}
return sessionFactory;
}
}
Create StudentDao Class
Let's create a separate StudentDao class to separate out Hibernate and the database related stuff.
package net.javaguides.hibernate.dao;
import java.util.List;
import org.hibernate.Session;
import org.hibernate.Transaction;
import net.javaguides.hibernate.entity.Student;
import net.javaguides.hibernate.util.HibernateUtil;
public class StudentDao {
public void saveStudent(Student student) {
Transaction transaction = null;
try (Session session = HibernateUtil.getSessionFactory().openSession()) {
// start a transaction
transaction = session.beginTransaction();
// save the student object
session.persist(student);
// commit transaction
transaction.commit();
} catch (Exception e) {
if (transaction != null) {
transaction.rollback();
}
e.printStackTrace();
}
}
public void updateStudent(Student student) {
Transaction transaction = null;
try (Session session = HibernateUtil.getSessionFactory().openSession()) {
// start a transaction
transaction = session.beginTransaction();
// save the student object
session.update(student);
// commit transaction
transaction.commit();
} catch (Exception e) {
if (transaction != null) {
transaction.rollback();
}
e.printStackTrace();
}
}
public void getStudent(int id) {
Transaction transaction = null;
try (Session session = HibernateUtil.getSessionFactory().openSession()) {
// start a transaction
transaction = session.beginTransaction();
// get Student entity using get() method
Student student = session.get(Student.class, id);
System.out.println(student.getFirstName());
System.out.println(student.getEmail());
// commit transaction
transaction.commit();
} catch (Exception e) {
if (transaction != null) {
transaction.rollback();
}
e.printStackTrace();
}
}
public void deleteStudent(int id) {
Transaction transaction = null;
try (Session session = HibernateUtil.getSessionFactory().openSession()) {
// start a transaction
transaction = session.beginTransaction();
// Delete a persistent object
Student student = session.get(Student.class, id);
if (student != null) {
session.delete(student);
System.out.println("student 1 is deleted");
}
// commit transaction
transaction.commit();
} catch (Exception e) {
if (transaction != null) {
transaction.rollback();
}
e.printStackTrace();
}
}
public List < Student > getStudents() {
try (Session session = HibernateUtil.getSessionFactory().openSession()) {
return session.createQuery("from Student", Student.class).list();
}
}
}
7. Create the main App class and Run an Application
Let's test Hibernate application to connect the MySQL database.
package net.javaguides.hibernate;
import java.util.List;
import net.javaguides.hibernate.dao.StudentDao;
import net.javaguides.hibernate.entity.Student;
public class App {
public static void main(String[] args) {
StudentDao studentDao = new StudentDao();
Student student = new Student("Ramesh", "Fadatare", "[email protected]");
studentDao.saveStudent(student);
List < Student > students = studentDao.getStudents();
students.forEach(s - > System.out.println(s.getFirstName()));
}
}
Output
GitHub Repository
The complete source code of this article is available on my GitHub Repository - https://github.com/RameshMF/Hibernate-ORM-Tutorials
Conclusion
In this article, we have shown how to use c3p0 connection pooling in hibernate applications.
You can learn more about Hibernate ORM Framework at Hibernate Tutorial
Hello, you don´t close connections. Why?
ReplyDelete