In the previous article, we have discussed reading XML file using DOM parser in Java. Now in this article, we will learn how to read XML file in Java using SAX parser.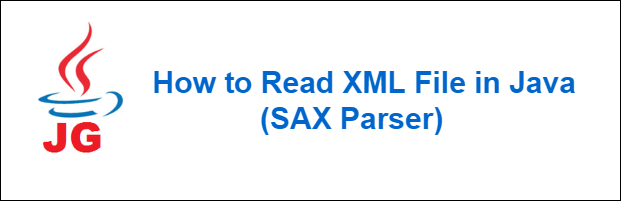
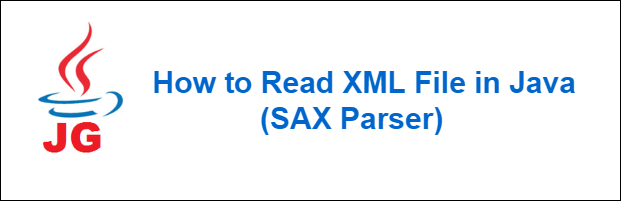
SAX parser is working differently with a DOM parser, it neither load any XML document into memory nor creates an object representation of the XML document. Instead, the SAX parser use callback function (org.xml.sax.helpers.DefaultHandler) to informs clients of the XML document structure.
SAX Parser is faster and uses less memory than DOM parser.
We need to use below SAX parser classes and interfaces to read XML file in Java.
- javax.xml.parsers.SAXParser provides a method to parse XML document using event handlers. This class implements XMLReader interface and provides overloaded versions of parse() methods to read XML document from File, InputStream, SAX InputSource, and String URI.
- The actual parsing is done by the Handler class. We need to create our own handler class to parse the XML document. We need to implement org.xml.sax.ContentHandler interface to create our own handler classes. This interface contains callback methods that receive notification when an event occurs. For example StartDocument, EndDocument, StartElement, EndElement, CharacterData etc.
- org.xml.sax.helpers.DefaultHandler provides a default implementation of the ContentHandler interface and we can extend this class to create our own handler. It’s advisable to extend this class because we might need only a few of the methods to implement. Extending this class will keep our code cleaner and maintainable.
Development Process Steps
- Input sax_users.xml File(XML file we will read)
- Create SAX Parser Handler class extending DefaultHandler class
- Create User class (populate XML data into User object)
- Program to Read XML File in Java using Java DOM Parser
1. Input users.xml File(XML file we will read)
Here is the XML file that will be read in this program.
<?xml version="1.0" encoding="UTF-8"?>
<Users>
<User id= "1">
<firstName>Ramesh</firstName>
<lastName>Fadatare</lastName>
<age>28</age>
<gender>Male</gender>
</User>
<User id= "2">
<firstName>John</firstName>
<lastName>Cena</lastName>
<age>45</age>
<gender>Male</gender>
</User>
<User id= "3">
<firstName>Tom</firstName>
<lastName>Cruise</lastName>
<age>40</age>
<gender>Male</gender>
</User>
</Users>
2. Create SAX Parser Handler class extending DefaultHandler class
Let’s create our own SAX Parser Handler class extending DefaultHandler class.
package net.javaguides.javaxmlparser.sax;
import java.util.ArrayList;
import java.util.List;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
public class UserHandler extends DefaultHandler {
private boolean hasFirstName = false;
private boolean hasLastName = false;
private boolean hasAge = false;
private boolean hasGender = false;
// List to hold Users object
private List < User > userList = null;
private User user = null;
// getter method for userloyee list
public List < User > getEmpList() {
return userList;
}
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
if (qName.equalsIgnoreCase("User")) {
// create a new User and put it in Map
String id = attributes.getValue("id");
// initialize User object and set id attribute
user = new User();
user.setId(Integer.parseInt(id));
// initialize list
if (userList == null)
userList = new ArrayList < > ();
} else if (qName.equalsIgnoreCase("firstName")) {
// set boolean values for fields, will be used in setting User variables
hasFirstName = true;
} else if (qName.equalsIgnoreCase("age")) {
hasAge = true;
} else if (qName.equalsIgnoreCase("gender")) {
hasGender = true;
} else if (qName.equalsIgnoreCase("lastName")) {
hasLastName = true;
}
}
@Override
public void endElement(String uri, String localName, String qName) throws SAXException {
if (qName.equalsIgnoreCase("User")) {
// add User object to list
userList.add(user);
}
}
@Override
public void characters(char ch[], int start, int length) throws SAXException {
if (hasAge) {
// age element, set User age
user.setAge(Integer.parseInt(new String(ch, start, length)));
hasAge = false;
} else if (hasFirstName) {
user.setFirstName(new String(ch, start, length));
hasFirstName = false;
} else if (hasLastName) {
user.setLastName(new String(ch, start, length));
hasLastName = false;
} else if (hasGender) {
user.setGender(new String(ch, start, length));
hasGender = false;
}
}
}
3. Create User class (populate XML data into User object)
So this XML is the list of users, to read this XML file we will create a bean object User and then we will parse the XML to get the list of users.
Here is the User bean object.
package net.javaguides.javaxmlparser.sax;
public class User {
private int id;
private String firstName;
private String lastName;
private int age;
private String gender;
public int getId() {
return id;
}
public void setId(int i) {
this.id = i;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
@Override
public String toString() {
return "User [id=" + id + ", firstName=" + firstName + ", lastName=" + lastName + ", age=" + age + ", gender=" +
gender + "]";
}
}
4. Program to Read XML File in Java using Java SAX Parser
package net.javaguides.javaxmlparser.sax;
import java.io.File;
import java.io.IOException;
import java.util.List;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import org.xml.sax.SAXException;
public class ReadXMLSAXParser {
public static void main(String[] args) {
SAXParserFactory saxParserFactory = SAXParserFactory.newInstance();
try {
SAXParser saxParser = saxParserFactory.newSAXParser();
UserHandler handler = new UserHandler();
saxParser.parse(new File("sax_users.xml"), handler);
// Get Users list
List < User > userList = handler.getEmpList();
// print user information
for (User user: userList)
System.out.println(user);
} catch (ParserConfigurationException | SAXException | IOException e) {
e.printStackTrace();
}
}
}
An output of above program is:
User [id=1, firstName=Ramesh, lastName=Fadatare, age=28, gender=Male]
User [id=2, firstName=John, lastName=Cena, age=45, gender=Male]
User [id=3, firstName=Tom, lastName=Cruise, age=40, gender=Male]
Comments
Post a Comment
Leave Comment