In this tutorial, we will learn how to develop CRUD RESTFul API using Spring Boot, Hibernate, JPA, Maven, and MySQL database.
In this tutorial, we will use the latest version of Spring Boot 3, Hibernate 6, and Java 17.
Source Code on GitHub
This tutorial is accompanied by a working code example on GitHub.YouTube Video
This tutorial is explained in below Youtube Video:
Subscribe to my youtube channel here at Java Guides - YouTube Channel
Use the following details while creating the Spring Boot project:
All your domain models must be annotated with @Entity annotation. It is used to mark the class as a persistent Java class.
@Table annotation is used to provide the details of the table that this entity will be mapped to.
@Id annotation is used to define the primary key.
@GeneratedValue annotation is used to define the primary key generation strategy. In the above case, we have declared the primary key to be an Auto Increment field.
@Column annotation is used to define the properties of the column that will be mapped to the annotated field. You can define several properties like name, length, nullable, updateable etc.
In the above code, the UserRepository interface extends JpaRepository which provides below methods to deal with database operations:
Let's understand all the annotations used in the UserController:
@RestController - annotation is a combination of Spring’s @Controller and @ResponseBody annotations.
@PostMapping - annotation is a short form of @RequestMapping(method=RequestMethod.POST). This annotation is used to map incoming HTTP POST requests to a specific method handler.
@PutMapping - annotation is a short form of @RequestMapping(method=RequestMethod.PUT).This annotation is used to map incoming HTTP PUT requests to a specific method handler.
@DeleteMapping - annotation is a short form of @RequestMapping(method=RequestMethod.DELETE). This annotation is used to map incoming HTTP DELETE requests to a specific method handler.
@PathVariable - annotation is used to bind a path variable with a method parameter.
From the above Spring Boot application development let's list out the advantages of using Spring boot.
1. What we’ll build
We are building a simple User Management Application which has below CRUD Rest APIs.We are going to build the following five REST APIs (Controller handler methods) for the User resource.
1. Creating Spring Boot Project
There are many ways to create a Spring Boot application. The simplest way is to use Spring Initializr at http://start.spring.io/, which is an online Spring Boot application generator.
Use the following details while creating the Spring Boot project:
- Generate: Maven Project
- Java Version: 17 (Default)
- Spring Boot:3.0.4
- Group: com.companyname
- Artifact: springbootcrudrest
- Name: springbootcrudrest
- Description: Rest API for a Simple User Management Application
- Package Name : com.companyname.springbootcrudrest
- Packaging: jar (This is the default value)
- Dependencies: Web, JPA, MySQL, DevTools
3. Maven Dependencies - pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.companyname</groupId>
<artifactId>springbootcrudrest</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>springbootcrudrest</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.0.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>com.mysql</groupId>
<artifactId>mysql-connector-j</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
From the above pom.xml, let's understand a few important spring boot features.
Spring Boot Maven plugin
The Spring Boot Maven plugin provides many convenient features:- It collects all the jars on the classpath and builds a single, runnable "über-jar", which makes it more convenient to execute and transport your service.
- It searches for the public static void main() method to flag as a runnable class.
- It provides a built-in dependency resolver that sets the version number to match Spring Boot dependencies. You can override any version you wish, but it will default to Boot’s chosen set of versions.
spring-boot-starter-parent
All Spring Boot projects typically use spring-boot-starter-parent as the parent in pom.xml. <parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.0.4</version>
</parent>
The spring-boot-starter-parent allows you to manage the following things for multiple child projects and modules:
- Configuration - Java Version and Other Properties
- Dependency Management - Version of dependencies
- Default Plugin Configuration
spring-boot-starter-web
We added the spring-boot-starter-web dependency, which will by default pulls all the commonly used libraries while developing Spring MVC applications, such as spring-webmvc, jackson-json, validation-api, and Tomcat.
spring-boot-starter-data-jpa
We added the spring-boot-starter-data-jpa dependency. This pulls all the spring-data-jpa dependencies and adds Hibernate libraries because most applications use Hibernate as a JPA implementation.3. Configuring MySQL Database
Since we’re using MySQL as our database, we need to configure the database URL, username, and password so that Spring can establish a connection with the database on startup.Open the src/main/resources/application.properties file and add the following properties to it -
spring.datasource.url = jdbc:mysql://localhost:3306/users_database?useSSL=false spring.datasource.username = root spring.datasource.password = root ## Hibernate Properties # The SQL dialect makes Hibernate generate better SQL for the chosen database spring.jpa.properties.hibernate.dialect = org.hibernate.dialect.MySQLDialect # Hibernate ddl auto (create, create-drop, validate, update) spring.jpa.hibernate.ddl-auto = update
Don’t forget to change the spring.datasource.username and spring.datasource.password as per your MySQL installation. Also, create a database named users_database in MySQL before proceeding to the next section.
You don’t need to create any tables. The tables will automatically be created by hibernate from the User entity that we will define in the next step. This is made possible by the property spring.jpa.hibernate.ddl-auto = update.
You don’t need to create any tables. The tables will automatically be created by hibernate from the User entity that we will define in the next step. This is made possible by the property spring.jpa.hibernate.ddl-auto = update.
4. Create a JPA Entity - User
Let's create a User model or domain class with the following fields:
- id - primary key
- firstName - user's first name
- lastName - user last name
- emailId - user email ID
- createdAt - user object created date
- createdBy - use an object created by
- updatedAt - user object updated by
- updatedby - user object updated by
package com.companyname.springbootcrudrest.model; import java.util.Date; import jakarta.persistence.*; import org.springframework.data.annotation.CreatedBy; import org.springframework.data.annotation.CreatedDate; import org.springframework.data.annotation.LastModifiedBy; import org.springframework.data.annotation.LastModifiedDate; import org.springframework.data.jpa.domain.support.AuditingEntityListener; @Entity @Table(name = "users") @EntityListeners(AuditingEntityListener.class) public class User { private long id; private String firstName; private String lastName; private String emailId; private Date createdAt; private String createdBy; private Date updatedAt; private String updatedby; @Id @GeneratedValue(strategy = GenerationType.AUTO) public long getId() { return id; } public void setId(long id) { this.id = id; } @Column(name = "first_name", nullable = false) public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } @Column(name = "last_name", nullable = false) public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } @Column(name = "email_address", nullable = false) public String getEmailId() { return emailId; } public void setEmailId(String emailId) { this.emailId = emailId; } @Column(name = "created_at", nullable = false) @CreatedDate public Date getCreatedAt() { return createdAt; } public void setCreatedAt(Date createdAt) { this.createdAt = createdAt; } @Column(name = "created_by", nullable = false) @CreatedBy public String getCreatedBy() { return createdBy; } public void setCreatedBy(String createdBy) { this.createdBy = createdBy; } @Column(name = "updated_at", nullable = false) @LastModifiedDate public Date getUpdatedAt() { return updatedAt; } public void setUpdatedAt(Date updatedAt) { this.updatedAt = updatedAt; } @Column(name = "updated_by", nullable = false) @LastModifiedBy public String getUpdatedby() { return updatedby; } public void setUpdatedby(String updatedby) { this.updatedby = updatedby; } }
All your domain models must be annotated with @Entity annotation. It is used to mark the class as a persistent Java class.
@Table annotation is used to provide the details of the table that this entity will be mapped to.
@Id annotation is used to define the primary key.
@GeneratedValue annotation is used to define the primary key generation strategy. In the above case, we have declared the primary key to be an Auto Increment field.
@Column annotation is used to define the properties of the column that will be mapped to the annotated field. You can define several properties like name, length, nullable, updateable etc.
How to enable JPA Auditing
Let's understand important JPA Auditing annotations:- @CreatedDate - Declares a field as the one representing the date the entity containing the field was created.
- @LastModifiedDate - Declares a field as the one representing the date the entity containing the field was recently modified.
- @CreatedBy- Declares a field as the one representing the principal that created the entity containing the field.
- @LastModifiedBy - Declares a field as the one representing the principal that recently modified the entity containing the field.
To achieve this, we need to do two things -
1. Add Spring Data JPA’s AuditingEntityListener to the domain model. We have already done this in our User model with the annotation @EntityListeners(AuditingEntityListener.class).
2. Enable JPA Auditing in the main application.
Open SpringBootCrudRestApplication.java and add a @EnableJpaAuditing annotation.
So far we have created a User model and enabled JPA auditing on it. Next, we need to create a repository to access user records to and from a database.
1. Add Spring Data JPA’s AuditingEntityListener to the domain model. We have already done this in our User model with the annotation @EntityListeners(AuditingEntityListener.class).
@Entity
@Table(name = "users")
@EntityListeners(AuditingEntityListener.class)
public class User {
// rest of the code here
}
Open SpringBootCrudRestApplication.java and add a @EnableJpaAuditing annotation.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.data.jpa.repository.config.EnableJpaAuditing;
@SpringBootApplication
@EnableJpaAuditing
public class SpringBootCrudRestApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootCrudRestApplication.class, args);
}
}
5. Create Spring Data JPA Repository - UserRepository
import org.springframework.data.jpa.repository.JpaRepository;
import com.companyname.springbootcrudrest.model.User;
public interface UserRepository extends JpaRepository<User, Long>{
}
List<T> findAll();
List<T> findAll(Sort sort);
List<T> findAllById(Iterable<ID> ids);
<S extends T> List<S> saveAll(Iterable<S> entities);
void flush();
<S extends T> S saveAndFlush(S entity);
void deleteInBatch(Iterable<T> entities);
void deleteAllInBatch();
T getOne(ID id);
@Override
<S extends T> List<S> findAll(Example<S> example);
<S extends T> List<S> findAll(Example<S> example, Sort sort);
6. Exception(Error) Handling for RESTful Services
ResourceNotFoundException
Let's create a ResourceNotFoundException class with the following content in it:
package com.companyname.springbootcrudrest.exception;
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ResponseStatus;
@ResponseStatus(value = HttpStatus.NOT_FOUND)
public class ResourceNotFoundException extends Exception{
private static final long serialVersionUID = 1L;
public ResourceNotFoundException(String message){
super(message);
}
}
ErrorDetails
Let's create ErrorDetails that we will use to customize the error response structure:package com.companyname.springbootcrudrest.exception;
import java.util.Date;
public class ErrorDetails {
private Date timestamp;
private String message;
private String details;
public ErrorDetails(Date timestamp, String message, String details) {
super();
this.timestamp = timestamp;
this.message = message;
this.details = details;
}
public Date getTimestamp() {
return timestamp;
}
public String getMessage() {
return message;
}
public String getDetails() {
return details;
}
}
GlobalExceptionHandler
To use ErrorDetails to return the error response, let’s create a GlobalExceptionHandler class annotated with @ControllerAdvice annotation. This class handles exception-specific and global exceptions in a single place.package com.companyname.springbootcrudrest.exception;
import java.util.Date;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.context.request.WebRequest;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<?> resourceNotFoundException(ResourceNotFoundException ex, WebRequest request) {
ErrorDetails errorDetails = new ErrorDetails(new Date(), ex.getMessage(), request.getDescription(false));
return new ResponseEntity<>(errorDetails, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<?> globleExcpetionHandler(Exception ex, WebRequest request) {
ErrorDetails errorDetails = new ErrorDetails(new Date(), ex.getMessage(), request.getDescription(false));
return new ResponseEntity<>(errorDetails, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
7. Creating UserController(Contains REST APIs)
Now, it's time to create CRUD Rest APIs for the User model.package com.companyname.springbootcrudrest.controller;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import jakarta.validation.Valid;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.companyname.springbootcrudrest.exception.ResourceNotFoundException;
import com.companyname.springbootcrudrest.model.User;
import com.companyname.springbootcrudrest.repository.UserRepository;
@RestController
@RequestMapping("/api/v1")
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping("/users")
public List<User> getAllUsers() {
return userRepository.findAll();
}
@GetMapping("/users/{id}")
public ResponseEntity<User> getUserById(
@PathVariable(value = "id") Long userId) throws ResourceNotFoundException {
User user = userRepository.findById(userId)
.orElseThrow(() -> new ResourceNotFoundException("User not found on :: "+ userId));
return ResponseEntity.ok().body(user);
}
@PostMapping("/users")
public User createUser(@Valid @RequestBody User user) {
return userRepository.save(user);
}
@PutMapping("/users/{id}")
public ResponseEntity<User> updateUser(
@PathVariable(value = "id") Long userId,
@Valid @RequestBody User userDetails) throws ResourceNotFoundException {
User user = userRepository.findById(userId)
.orElseThrow(() -> new ResourceNotFoundException("User not found on :: "+ userId));
user.setEmailId(userDetails.getEmailId());
user.setLastName(userDetails.getLastName());
user.setFirstName(userDetails.getFirstName());
user.setUpdatedAt(new Date());
final User updatedUser = userRepository.save(user);
return ResponseEntity.ok(updatedUser);
}
@DeleteMapping("/user/{id}")
public Map<String, Boolean> deleteUser(
@PathVariable(value = "id") Long userId) throws Exception {
User user = userRepository.findById(userId)
.orElseThrow(() -> new ResourceNotFoundException("User not found on :: "+ userId));
userRepository.delete(user);
Map<String, Boolean> response = new HashMap<>();
response.put("deleted", Boolean.TRUE);
return response;
}
}
@RequestMapping("/api/v1") - annotation declares that the URL for all the APIs in this controller will start with /api/v1
@RestController - annotation is a combination of Spring’s @Controller and @ResponseBody annotations.
@GetMapping - annotation is a short form of @RequestMapping(method=RequestMethod.GET). This annotation is used to map incoming HTTP GET requests to a specific method handler.
@PostMapping - annotation is a short form of @RequestMapping(method=RequestMethod.POST). This annotation is used to map incoming HTTP POST requests to a specific method handler.
@PutMapping - annotation is a short form of @RequestMapping(method=RequestMethod.PUT).This annotation is used to map incoming HTTP PUT requests to a specific method handler.
@DeleteMapping - annotation is a short form of @RequestMapping(method=RequestMethod.DELETE). This annotation is used to map incoming HTTP DELETE requests to a specific method handler.
@PathVariable - annotation is used to bind a path variable with a method parameter.
8. Running the Application
We have successfully developed all the CRUD Rest APIs for the User model. now it's time to deploy our application in a servlet container(embedded tomcat). Two ways we can start the standalone Spring boot application.1. From the root directory of the application and type the following command to run it -
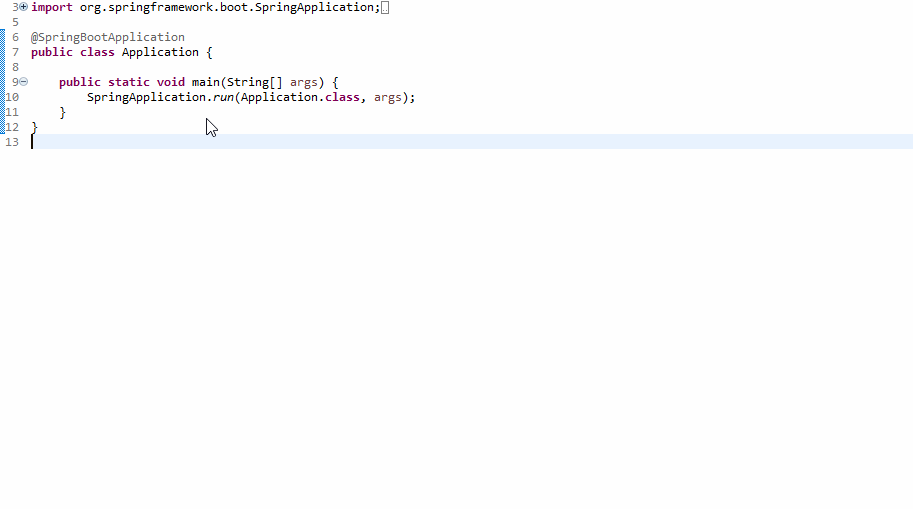
$ mvn spring-boot:run
2. From your IDE, run the SpringBootCrudRestApplication.main() method as a standalone Java class that will start the embedded Tomcat server on port 8080 and point the browser to http://localhost:8080/.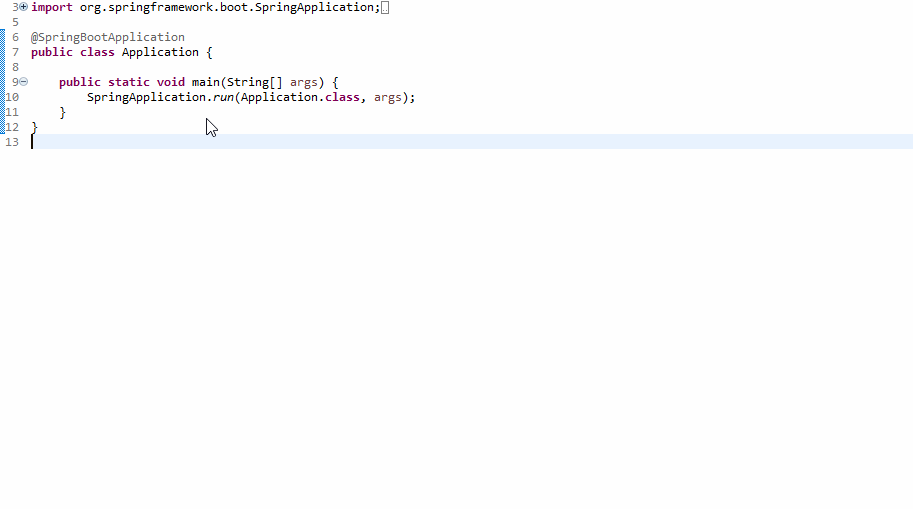
9. Unit Testing REST APIs
Let's write JUnit test cases for all Rest APIs of the User entity.package com.companyname.projectname.springbootcrudrest;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNotNull;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.boot.test.web.client.TestRestTemplate;
import org.springframework.boot.web.server.LocalServerPort;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.web.client.HttpClientErrorException;
import com.companyname.springbootcrudrest.SpringBootCrudRestApplication;
import com.companyname.springbootcrudrest.model.User;
@RunWith(SpringRunner.class)
@SpringBootTest(classes = SpringBootCrudRestApplication.class, webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class SpringBootCrudRestApplicationTests {
@Autowired
private TestRestTemplate restTemplate;
@LocalServerPort
private int port;
private String getRootUrl() {
return "http://localhost:" + port;
}
@Test
public void contextLoads() {
}
@Test
public void testGetAllUsers() {
HttpHeaders headers = new HttpHeaders();
HttpEntity<String> entity = new HttpEntity<String>(null, headers);
ResponseEntity<String> response = restTemplate.exchange(getRootUrl() + "/users",
HttpMethod.GET, entity, String.class);
assertNotNull(response.getBody());
}
@Test
public void testGetUserById() {
User user = restTemplate.getForObject(getRootUrl() + "/users/1", User.class);
System.out.println(user.getFirstName());
assertNotNull(user);
}
@Test
public void testCreateUser() {
User user = new User();
user.setEmailId("admin@gmail.com");
user.setFirstName("admin");
user.setLastName("admin");
user.setCreatedBy("admin");
user.setUpdatedby("admin");
ResponseEntity<User> postResponse = restTemplate.postForEntity(getRootUrl() + "/users", user, User.class);
assertNotNull(postResponse);
assertNotNull(postResponse.getBody());
}
@Test
public void testUpdatePost() {
int id = 1;
User user = restTemplate.getForObject(getRootUrl() + "/users/" + id, User.class);
user.setFirstName("admin1");
user.setLastName("admin2");
restTemplate.put(getRootUrl() + "/users/" + id, user);
User updatedUser = restTemplate.getForObject(getRootUrl() + "/users/" + id, User.class);
assertNotNull(updatedUser);
}
@Test
public void testDeletePost() {
int id = 2;
User user = restTemplate.getForObject(getRootUrl() + "/users/" + id, User.class);
assertNotNull(user);
restTemplate.delete(getRootUrl() + "/users/" + id);
try {
user = restTemplate.getForObject(getRootUrl() + "/users/" + id, User.class);
} catch (final HttpClientErrorException e) {
assertEquals(e.getStatusCode(), HttpStatus.NOT_FOUND);
}
}
}
Output
From the above Spring Boot application development let's list out the advantages of using Spring boot.
- Simpler dependency management
- Default auto-configuration
- Embedded web server
- Application metrics and health checks
- Advanced externalized configuration
10. Test Using Postman Client
1. Create User REST API
{
"firstName": "Ramesh",
"lastName": "fadatare",
"emailId": "ramesh@gmail.com",
"createdBy": "Ramesh",
"updatedby": "Ramesh"
}
2. Get User by ID REST API
HTTP Method: GET
Request URL: http://localhost:8080/api/v1/users/2
Request URL: http://localhost:8080/api/v1/users/2
3. Get all users REST API
HTTP Method: GET
Request URL: http://localhost:8080/api/v1/users
Request URL: http://localhost:8080/api/v1/users
4. Update User REST API
HTTP Method: GET
Request URL: http://localhost:8080/api/v1/users/5
Request JSON:
{
"firstName": "Ram",
"lastName": "Jadhav",
"emailId": "ramesh123@gmail.com",
"createdBy": "Ramesh",
"updatedby": "Ramesh"
}
5. Delete User REST API
HTTP Method: DELETE
Request URL: http://localhost:8080/api/v1/users/2
12. Source code on GitHub
The source code of this tutorial is available on my GitHub Repository.
I ran this project in IntelliJ and it is giving me following error:
ReplyDeleteError:(16, 43) java: diamond operator is not supported in -source 1.5
(use -source 7 or higher to enable diamond operator)
How do I change this?
Thank you very much.
You should change jdk 1.5 to 1.8. Google it how to configure jdk 1.8 in intellij.
ReplyDeletehere table will be auto created or not?
ReplyDeleteYes, tables will be automatically created as you can see the ddl-auto configuration in application.properties file.
ReplyDeletei dont know ,why table is not creating for me
Deleteyes.. table will be auto created but not database. you need to create a database first.
DeleteI am new with Springboot. I don't even quite get why we use localhost:8080 when using postman to crud our records instead of localhost:3306? I was thinking because we are connecting those records to mysql database and our records should be visible in mysql database, but I couldn't see the update through dbeaver? Thank you.
ReplyDeleteGreat tutorial, but I have this error while I run the test, like this:
ReplyDeleteava.lang.IllegalStateException: Failed to load ApplicationContext
at org.springframework.test.context.cache.DefaultCacheAwareContextLoaderDelegate.loadContext(DefaultCacheAwareContextLoaderDelegate.java:132)
at org.springframework.test.context.support.DefaultTestContext.getApplicationContext(DefaultTestContext.java:123)
at org.springframework.test.context.support.DependencyInjectionTestExecutionListener.injectDependencies(DependencyInjectionTestExecutionListener.java:118)
at org.springframework.test.context.support.DependencyInjectionTestExecutionListener.prepareTestInstance(DependencyInjectionTestExecutionListener.java:83)
at org.springframework.boot.test.autoconfigure.SpringBootDependencyInjectionTestExecutionListener.prepareTestInstance(SpringBootDependencyInjectionTestExecutionListener.java:43)
at org.springframework.test.context.TestContextManager.prepareTestInstance(TestContextManager.java:244)
at org.springframework.test.context.junit.jupiter.SpringExtension.postProcessTestInstance(SpringExtension.java:98)
at org.junit.jupiter.engine.descriptor.ClassBasedTestDescriptor.lambda$invokeTestInstancePostProcessors$5(ClassBasedTestDescriptor.java:341)
at org.junit.jupiter.engine.descriptor.ClassBasedTestDescriptor.executeAndMaskThrowable(ClassBasedTestDescriptor.java:346)
at org.junit.jupiter.engine.descriptor.ClassBasedTestDescriptor.lambda$invokeTestInstancePostProcessors$6(ClassBasedTestDescriptor.java:341)
at java.base/java.util.stream.ReferencePipeline$3$1.accept(ReferencePipeline.java:195)
at java.base/java.util.stream.ReferencePipeline$2$1.accept(ReferencePipeline.java:177)
at java.base/java.util.ArrayList$ArrayListSpliterator.forEachRemaining(ArrayList.java:1624)
at java.base/java.util.stream.AbstractPipeline.copyInto(AbstractPipeline.java:484)
at java.base/java.util.stream.AbstractPipeline.wrapAndCopyInto(AbstractPipeline.java:474)
at java.base/java.util.stream.StreamSpliterators$WrappingSpliterator.forEachRemaining(StreamSpliterators.java:312)
at java.base/java.util.stream.Streams$ConcatSpliterator.forEachRemaining(Streams.java:735)
at java.base/java.util.stream.Streams$ConcatSpliterator.forEachRemaining(Streams.java:734)
I tried to put this on build tag:
src/test/resources
...
Is there any solution for that scenery? Thanks in advance!
I ran the application but while doing the post, it shows 404. plz helpme out
Delete{
"timestamp": "2020-10-27T15:17:43.739+00:00",
"status": 404,
"error": "Not Found",
"message": "",
"path": "/api/v1/users"
}
I rearranged the main class, i moved it to the top of the package and now it works...
Delete